WebGL Experiments
http://www.chromeexperiments.com/webgl
Learning WebGL
http://learningwebgl.com/blog/?page_id=1217
WebGL Specification
http://www.khronos.org/registry/webgl/specs/latest/
Problems & Solutions:
Problem 1: ‘Can not Initialise’ in Firefox 4+
1. Type “about:config” in your browser’s URL bar
2. Filter by ‘webgl’
3. Set webgl.force-enabled = true (double-click)
4. Set webgl.verbose = true (to see error Tools > Error Console)
5. Restart Firefox
http://support.mozilla.com/en-US/questions/720087
Problem 2: Cross-domain WebGL Texture doesn't render
- ‘forbidden to load a WebGL texture from a cross-domain element’ in Firefox 4+
- or 'Uncaught Error: SECURITY_ERR: DOM Exception 18' in Chrome 10+
Solution1: work locally
1. Encode the image into base64
Binary File to Base64 Encoder
http://www.greywyvern.com/code/php/binary2base64
2. Put data source in place of the image's path
<img src="data:image/jpeg;base64,iVBORw0KGgoAAAANSUhEUgAAADIA...really long string" alt="" />
http://www.devirtuoso.com/2011/09/working-with-images-and-html5-canvas-locally/
Solution2:
http://mmmovania.blogspot.com/2011/09/resolving-webgl-cross-domain-image.html
Tuesday, September 27, 2011
Friday, September 23, 2011
HTML 5, CSS3 and JavaScript
1. 8 Simply Amazing HTML5 Canvas and Javascript Animations
http://www.queness.com/post/3885/8-simply-amazing-html5-canvas-and-javascript-animations
e.g. Bomomo
2. 10 Jaw Dropping HTML5 and Javascript Effects
http://www.queness.com/post/4650/10-jaw-dropping-html5-and-javascript-effects
e.g. Blob
3. 13 Amazing Examples of HTML5 and CSS3
http://www.queness.com/post/4105/13-amazing-examples-of-html5-and-css3
e.g Coke Can
4. WebGL
http://www.chromeexperiments.com/webgl
http://www.queness.com/post/3885/8-simply-amazing-html5-canvas-and-javascript-animations
e.g. Bomomo
2. 10 Jaw Dropping HTML5 and Javascript Effects
http://www.queness.com/post/4650/10-jaw-dropping-html5-and-javascript-effects
e.g. Blob
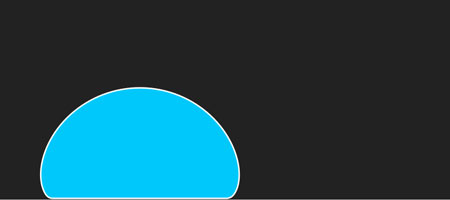
3. 13 Amazing Examples of HTML5 and CSS3
http://www.queness.com/post/4105/13-amazing-examples-of-html5-and-css3
e.g Coke Can
4. WebGL
http://www.chromeexperiments.com/webgl
how to prevent SQL injection
SQL Injection Attacks and Some Tips
http://www.codeproject.com/KB/database/SqlInjectionAttacks.aspx
- the initial apostrophe closes the opening quote in the original SQL statement.
- the two dashes at the end starts a comment, which means that anything left in the original SQL statement is ignored.
SQL Injection
http://en.wikipedia.org/wiki/SQL_injection
If this code were to be used in an authentication procedure then this example could be used to force the selection of a valid username because the evaluation of '1'='1' is always true.
http://www.wwwcoder.com/main/parentid/258/site/2966/68/default.aspx
http://www.codeproject.com/KB/database/SqlInjectionAttacks.aspx
' UNION SELECT name, type, id FROM sysobjects;--
- the initial apostrophe closes the opening quote in the original SQL statement.
- the two dashes at the end starts a comment, which means that anything left in the original SQL statement is ignored.
SQL Injection
http://en.wikipedia.org/wiki/SQL_injection
' or '1'='1
' or '1'='1' -- '
' or '1'='1' ({ '
' or '1'='1' /* '
If this code were to be used in an authentication procedure then this example could be used to force the selection of a valid username because the evaluation of '1'='1' is always true.
http://www.wwwcoder.com/main/parentid/258/site/2966/68/default.aspx
Tuesday, September 20, 2011
PHP mail()
No email was sent
1. check if sendmail server is running
1. check if sendmail server is running
[root]# service sendmail status
Configuring Linux Mail Servers
http://www.linuxhomenetworking.com/wiki/index.php/Quick_HOWTO_:_Ch21_:_Configuring_Linux_Mail_Servers#Starting_Sendmail
Friday, September 2, 2011
Prevent Cross Site Scripting
1. HTML and JavaScript
http://www.codeproject.com/KB/web-security/Security_HTML_Injection.aspx
2. PHP: Preventing typical XSS attacks
http://chriscook.me/web-development/php-preventing-typical-xss-attacks/
3. 15 PHP regular expressions for web developers
http://www.catswhocode.com/blog/15-php-regular-expressions-for-web-developers
4. XSS (Cross Site Scripting) Prevention Cheat Sheet
https://www.owasp.org/index.php/XSS_%28Cross_Site_Scripting%29_Prevention_Cheat_Sheet#Why_Can.27t_I_Just_HTML_Entity_Encode_Untrusted_Data.3F
5. PHP Regular Expression
http://php-regex.blogspot.com/2008/01/introduction-to-regular-expressions-in.html
6. Using Regular Expressions with PHP
http://www.webcheatsheet.com/php/regular_expressions.php
7. Regular Expression Basic Syntax Reference
http://www.regular-expressions.info/reference.html
8. Using a Regular Expression to Match HTML
http://haacked.com/archive/2004/10/25/usingregularexpressionstomatchhtml.aspx
9 Ultimate Regular Expression for HTML tag parsing with PHP
http://kevin.deldycke.com/2007/03/ultimate-regular-expression-for-html-tag-parsing-with-php/
Literal Text:
- The characters that match themselves are called literals
Metacharacter:
If you want to match a literal metacharacter in a pattern, you have to escape it with a backslash.
http://www.codeproject.com/KB/web-security/Security_HTML_Injection.aspx
2. PHP: Preventing typical XSS attacks
http://chriscook.me/web-development/php-preventing-typical-xss-attacks/
3. 15 PHP regular expressions for web developers
http://www.catswhocode.com/blog/15-php-regular-expressions-for-web-developers
4. XSS (Cross Site Scripting) Prevention Cheat Sheet
https://www.owasp.org/index.php/XSS_%28Cross_Site_Scripting%29_Prevention_Cheat_Sheet#Why_Can.27t_I_Just_HTML_Entity_Encode_Untrusted_Data.3F
5. PHP Regular Expression
http://php-regex.blogspot.com/2008/01/introduction-to-regular-expressions-in.html
6. Using Regular Expressions with PHP
http://www.webcheatsheet.com/php/regular_expressions.php
7. Regular Expression Basic Syntax Reference
http://www.regular-expressions.info/reference.html
8. Using a Regular Expression to Match HTML
http://haacked.com/archive/2004/10/25/usingregularexpressionstomatchhtml.aspx
9 Ultimate Regular Expression for HTML tag parsing with PHP
http://kevin.deldycke.com/2007/03/ultimate-regular-expression-for-html-tag-parsing-with-php/
Literal Text:
- The characters that match themselves are called literals
Metacharacter:
- backslash \ :
- caret ^ : at the beginning of a regular expression indicates that it must match the beginning of the string
- dollar sign $ : match strings that end with the given pattern.
- period or dot . : matches any single character except newline (\). e.g. the pattern h.t matches hat, hothit, hut, h7t, etc
- vertical bar or pipe symbol | : is used for alternatives in a regular expression.
- question mark ? :
- asterisk or star * :
- plus sign + :
- square bracket [ ] :
- round bracket ( ) :
- brace { } :
If you want to match a literal metacharacter in a pattern, you have to escape it with a backslash.
[agk] matches any one a, g, or k [a-z] matches any one character from a to z [^z] matches any character other than z [\\(\\)] matches ( or ) (in javascript, the escape slash must be escaped!) . any character except \n \w any word character, same as [a-zA-Z0-9_] \W any non-word character \s any whitespace character, same as [ \t\n\r\f\v] \S any non-whitespace character \d any digit \D any non-digit \/ literal / \\ literal \ \. literal . \* literal * \+ literal + \? literal ? \| literal | \( literal ( \) literal ) \[ literal [ \] literal ] \- the - must be escaped inside brackets: [a-z0-9 _.\-\?!] {n,m} match previous item n to m times {n,} match previous item n or more times {n} match exactly n times ? match zero or once, same as {0,1}, also makes + and * "lazy" + match one or more * match zero or more | or (x|y) match x or y, inclusive (all x and y will be replaced) ( ) grouping and reference \1 reference to first grouping, used in the expression $1 reference to first grouping, used in the replacement string $$ literal $ used in the replacement string ^ anchor to the beginning of the string $ anchor to the end of the string \b match a word boundary (does not include the boundary) \B match a non word boundary (does not include the boundary) q(?=u) match q only before u (does not match the u) q(?!u) match q except before u i case-insensitive search, used like /expression/i g global replacement, used like /expression/g
Subscribe to:
Posts (Atom)